Do you actually need the show rule? If the only thing you want to do is to attach a footnote to the caption, there is another way.
First try
#let fig(
caption: [],
source: [],
show-source: true,
..args,
fig
) = {
let kind = args.named().at("kind", default: none)
let fn = if not show-source {
none
} else if source == [] and kind == "i-figured-image" {
footnote([Quelle: Eigene Darstellung])
} else {
footnote([Quelle: #source])
}
figure(
caption: caption + fn,
..args,
fig
)
}
#fig(
caption: "A rect with a source",
source: "The Typst rect function",
rect()
) <fig:rect>
#fig(
caption: "A rect without source",
show-source: false,
rect()
) <fig:rect2>
@fig:rect and @fig:rect2
For the time being this solution is not complete since you will find another bug, but this time related to the outline. When a footnote is attached to an outlined object the footnote is going to be repeated. In this comment of the related issue in GitHub there is a solution for this kind of problems. Given that, the full solution is this one
#set page(height: auto)
#let in-outline = state("in-outline", false)
#show outline: it => {
in-outline.update(true)
it
in-outline.update(false)
}
#let flex-caption(inside, outside) = context if in-outline.get() { inside } else { outside }
#let fig(
caption: [],
source: [],
show-source: true,
..args,
fig
) = {
let kind = args.named().at("kind", default: none)
let fn = if not show-source {
none
} else if source == [] and kind == "i-figured-image" {
footnote([Quelle: Eigene Darstellung])
} else {
footnote([Quelle: #source])
}
figure(
caption: flex-caption(caption, caption + fn),
..args,
fig
)
}
#outline(target: figure.where(kind:image))
#fig(
caption: "A rect with a source",
source: "The Typst rect function",
rect()
) <fig:rect>
#fig(
caption: "A rect without source",
show-source: false,
rect()
) <fig:rect2>
@fig:rect and @fig:rect2
Result
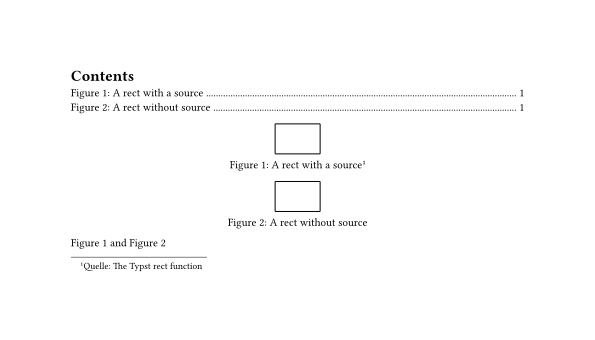
One last comment: in case you are using the show-source
argument just to avoid the footnote when the source is an empty content
, I suggest to get rid of that and add another branch in the if
statement to avoid the creation of a footnote in that case.